Logic to find all roots of a quadratic equation. Based on the above formula let us write step by step descriptive logic to find roots of a quadratic equation. Input coefficients of quadratic equation from user. Store it in some variable say a, b and c. Find discriminant of the given equation, using formula discriminant = (b.b) - (4.a.c). Write a C program to find all roots of Quadratic equation using switch case. Logic to find all roots of quadratic equation using switch case in C program. Learn C programming, Data Structures tutorials, exercises, examples, programs, hacks, tips and tricks online.
C++ Compiler
- The terminology is wrong. You are finding solutions to a quadratic equation, either using the quadratic formula or completing the square. You are not 'solving the quadratic formula by completing the square' (although the quadratic formula is derived by completing the square on the general equation).
- Press c and then Enter to continue. C Done $./quadraticsolver Enter the coefficients a, b, c for equation in the form ax^ + bx + c = 0: Enter value for a: 9 Enter value for b: 24 Enter value for c: 2 The roots are: x1 = -0.0861142, x2 = -2.58055 Press c and then Enter to continue.
- The program is compiled using Dev-C 4.9.9.2 version installed on a Windows 7 64-bit PC. You may try other standard C compilers and the program will still work if you use the correct C libraries. Problem Definition. In this program, we solve the quadratic equation using the formula.
I have given here a C program to solve any Quadratic Equation. Quadratic equation is a second order of polynomial equation in a single variable.
x = [ -b +/- sqrt(b^2 - 4ac) ] / 2a
We have to find the value of (b*b - 4*a*c).
I've compiled a list of 100+ resources for Christian women that will help with every 100+ Amazing Resources For Christian Women For 2021 Well over 100 resources for Christian women on topics like Messianic Judaism, living a life of faith, blogging, marriage, parenting, ministry, prayer and more! Just come to jesus free christian resources & downloads youtube. Christian Resources & Free Downloads. Christian Starter-Kit E-Book PDF here Christian Starter-Kit read online here. Free Downloads of the Bible Download-1st read me file for instructions Have questions on how to download here Adobe Reader free download Need winzip download at. Who else wants some free Christian downloads? Download these free mp3 and other resources to grow in your walk with God! When we see God in a more healthy way as loving, faithful, just and know that He is everywhere we will realize that he does care and is always with us wanting to connect with us. This will draw us closer to Him.
- When it is greater than Zero, we will get two Real Solutions.
- When it is equal to zero, we will get one Real Solution.
- When it is less than Zero, we will get two Imaginary Solutions.
When there is an imaginary solutions, we have to use the factor i to represent imaginary part as it is a complex number.
Source Code
#include<math.h>
#include<stdio.h>
// quadratic equation is a second order of polynomial equation in a single variable
// x = [ -b +/- sqrt(b^2 - 4ac) ] / 2a
void SolveQuadratic(double a, double b, double c)
{
double sqrtpart = b*b - 4*a*c;
double x, x1, x2, img;
if(sqrtpart > 0)
{
x1 = (-b + sqrt(sqrtpart)) / (2 * a);
x2 = (-b - sqrt(sqrtpart)) / (2 * a);
printf('Two Real Solutions: %.4lf or %.4lfnn', x1, x2);
}
elseif(sqrtpart < 0)
{
sqrtpart = -sqrtpart;
x = -b / (2 * a);

img = sqrt(sqrtpart) / (2 * a);
printf('Two Imaginary Solutions: %.4lf + %.4lf i or %.4lf + %.4lf inn', x, img, x, img);
}
else
{
x = (-b + sqrt(sqrtpart)) / (2 * a);
printf('One Real Solution: %.4lfnn', x);
}
}
int main()
{
// 6x^2 + 11x - 35 = 0
SolveQuadratic(6, 11, -35);
// 5x^2 + 6x + 1 = 0
SolveQuadratic(5, 6, 1);
// 2x^2 + 4x + 2 = 0

SolveQuadratic(2, 4, 2);
// 5x^2 + 2x + 1 = 0
SolveQuadratic(5, 2, 1);
return 0;
}Output
Two Real Solutions: 1.6667 or -3.5000
Two Real Solutions: -0.2000 or -1.0000
One Real Solution: -1.0000
Two Imaginary Solutions: -0.2000 + 0.4000 i or -0.2000 + 0.4000 i
Press any key to continue . . .
Dev C++ Online
The C++ program to solve quadratic equations in standard form is a simple program based on the quadratic formula. Given the coefficients as input, it will solve the equation and output the roots of the equation.
This is a simple program is intended for intermediate level C++ programmers.
The program is compiled using Dev-C++ 4.9.9.2 version installed on a Windows 7 64-bit PC. You may try other standard C compilers and the program will still work if you use the correct C libraries.
Problem Definition
In this program, we solve the quadratic equation using the formula
When
Where a, b and c are coefficient of the equation which is in the standard form.
How to compute?
The steps to compute the quadratic equation is given below.
Step1:
The program request the input coefficient values a, b and c. When the user input the values, it will compute two terms t1 and t3 and an intermediate term t2.
Step2:
The function term2 ()
is called in step 2 and returned value of function is assigned to t2
. The term2 ()
function receives the coefficient values – a, b, c and compute the value for t2.
The term ()
function returns and assign value of b2 – 4ac
to t2
and it is useful in understanding the root of the quadratic equation.
For example,
If (t2 < 0), then the roots are not real
If (t2 0) then, there is exactly one root value.
If (t2 > 0) then there are two root values.
The above condition is checked and printed with output values. Now we need to compute the roots and display the output values.
Step3:
A term t3
is assigned value after taking square root of t2.
Step4:
Finally, we have t1 and t3 to compute two roots of a quadratic equation.
Then root1
and root
are calculated and printed immediately.

Flowchart – Program for Quadratic Equations
To understand flow of logic of this program, see the flowchart below.
Program Code – Program for Quadratic Equation
Output
The output of the above program is given below.
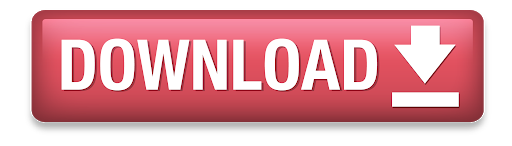